mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-21 07:07:30 +00:00
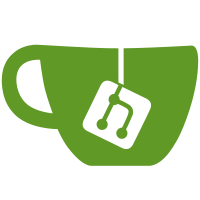
* initial sketch * add module migrations * add migrations for all accout types * test account migration * add tendermint migration and migrate cmd * remove need for errors pkg dependency * add bech32 decoding fork * add suggested params and cmd to write them * add basic upgrade instructions * fix tests * address some migration todos * tidy contrib folder * finalize params values * align cdp init genesis with other modules * add tendermint and distribution test add custom distribution migration to patch bug * add staking migration test * add slashing, evidence tests, refactor auth tests * add full migration test * remove go-amino dependency from go.mod also tidy up unused indirect dependencies * address remaining TODOs * remove commented out code from legacy types * add spot/liquidation markets ids to kava-3 params * Apply suggestions from code review Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> * address code review suggestions * add validate genesis to migrate test * refactor add params func * remove commented out code from old types * fix add params * add deputy address * add tests using exported kava-2 state * incorporate new cdp params from master * update params from review Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> * add deputy account * add committee permissions for new params Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com>
62 lines
1.8 KiB
Go
62 lines
1.8 KiB
Go
package v032
|
|
|
|
// ConsensusParams contains consensus critical parameters that determine the
|
|
// validity of blocks.
|
|
type ConsensusParams struct {
|
|
Block BlockParams `json:"block"`
|
|
Evidence EvidenceParams `json:"evidence"`
|
|
Validator ValidatorParams `json:"validator"`
|
|
}
|
|
|
|
// BlockParams define limits on the block size and gas plus minimum time
|
|
// between blocks.
|
|
type BlockParams struct {
|
|
MaxBytes int64 `json:"max_bytes"`
|
|
MaxGas int64 `json:"max_gas"`
|
|
// Minimum time increment between consecutive blocks (in milliseconds)
|
|
// Not exposed to the application.
|
|
TimeIotaMs int64 `json:"time_iota_ms"`
|
|
}
|
|
|
|
// EvidenceParams determine how we handle evidence of malfeasance.
|
|
type EvidenceParams struct {
|
|
MaxAge int64 `json:"max_age"` // only accept new evidence more recent than this
|
|
}
|
|
|
|
// ValidatorParams restrict the public key types validators can use.
|
|
// NOTE: uses ABCI pubkey naming, not Amino names.
|
|
type ValidatorParams struct {
|
|
PubKeyTypes []string `json:"pub_key_types"`
|
|
}
|
|
|
|
// // DefaultConsensusParams returns a default ConsensusParams.
|
|
func DefaultConsensusParams() *ConsensusParams {
|
|
return &ConsensusParams{
|
|
DefaultBlockParams(),
|
|
DefaultEvidenceParams(),
|
|
DefaultValidatorParams(),
|
|
}
|
|
}
|
|
|
|
// DefaultBlockParams returns a default BlockParams.
|
|
func DefaultBlockParams() BlockParams {
|
|
return BlockParams{
|
|
MaxBytes: 22020096, // 21MB
|
|
MaxGas: -1,
|
|
TimeIotaMs: 1000, // 1s
|
|
}
|
|
}
|
|
|
|
// DefaultEvidenceParams Params returns a default EvidenceParams.
|
|
func DefaultEvidenceParams() EvidenceParams {
|
|
return EvidenceParams{
|
|
MaxAge: 100000, // 27.8 hrs at 1block/s
|
|
}
|
|
}
|
|
|
|
// DefaultValidatorParams returns a default ValidatorParams, which allows
|
|
// only ed25519 pubkeys.
|
|
func DefaultValidatorParams() ValidatorParams {
|
|
return ValidatorParams{[]string{ABCIPubKeyTypeEd25519}}
|
|
}
|