mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
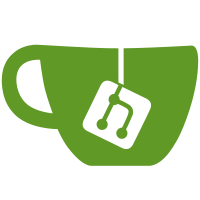
* start makefile refactor to smaller units; break out proto-dep updating; add check-proto-deps target for use in CI in order to determine if depdencies have diverged * add proto check workflow * download go modules before checking proto deps * clean up -- hide output and add error message for check target * add error message for check-rsync * update any type, and ibc-go protos for v3.4.0 * add buf generate files for gogo, docs, and swagger * update swagger dirs and run with latest swagger gen * ignore new build directories * refactor proto makefile logic -- use buf instead of scripts * remove old protobuf scripts * run all proto checks on push * remove moved file * set default value for protoc machine * install build deps seperately * fetch master for buf check breaking * checkout from https url in CI for buf breaking * fix rsync file permissions on darwin * ignore build dirs * fix issue with apple provided make; clean up build deps; switch to buf format * remove clang format file -- using buf format now * run make proto-format (buf format changes) * update generated files for proto format changes
81 lines
3.6 KiB
Protocol Buffer
81 lines
3.6 KiB
Protocol Buffer
syntax = "proto3";
|
|
package kava.router.v1beta1;
|
|
|
|
import "cosmos/base/v1beta1/coin.proto";
|
|
import "cosmos_proto/cosmos.proto";
|
|
import "gogoproto/gogo.proto";
|
|
|
|
option go_package = "github.com/kava-labs/kava/x/router/types";
|
|
option (gogoproto.goproto_getters_all) = false;
|
|
|
|
// Msg defines the router Msg service.
|
|
service Msg {
|
|
// MintDeposit converts a delegation into staking derivatives and deposits it all into an earn vault.
|
|
rpc MintDeposit(MsgMintDeposit) returns (MsgMintDepositResponse);
|
|
|
|
// DelegateMintDeposit delegates tokens to a validator, then converts them into staking derivatives,
|
|
// then deposits to an earn vault.
|
|
rpc DelegateMintDeposit(MsgDelegateMintDeposit) returns (MsgDelegateMintDepositResponse);
|
|
|
|
// WithdrawBurn removes staking derivatives from an earn vault and converts them back to a staking delegation.
|
|
rpc WithdrawBurn(MsgWithdrawBurn) returns (MsgWithdrawBurnResponse);
|
|
|
|
// WithdrawBurnUndelegate removes staking derivatives from an earn vault, converts them to a staking delegation,
|
|
// then undelegates them from their validator.
|
|
rpc WithdrawBurnUndelegate(MsgWithdrawBurnUndelegate) returns (MsgWithdrawBurnUndelegateResponse);
|
|
}
|
|
|
|
// MsgMintDeposit converts a delegation into staking derivatives and deposits it all into an earn vault.
|
|
message MsgMintDeposit {
|
|
// depositor represents the owner of the delegation to convert
|
|
string depositor = 1 [(cosmos_proto.scalar) = "cosmos.AddressString"];
|
|
// validator is the validator for the depositor's delegation
|
|
string validator = 2;
|
|
// amount is the delegation balance to convert
|
|
cosmos.base.v1beta1.Coin amount = 3 [(gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgMintDepositResponse defines the Msg/MsgMintDeposit response type.
|
|
message MsgMintDepositResponse {}
|
|
|
|
// MsgDelegateMintDeposit delegates tokens to a validator, then converts them into staking derivatives,
|
|
// then deposits to an earn vault.
|
|
message MsgDelegateMintDeposit {
|
|
// depositor represents the owner of the tokens to delegate
|
|
string depositor = 1 [(cosmos_proto.scalar) = "cosmos.AddressString"];
|
|
// validator is the address of the validator to delegate to
|
|
string validator = 2;
|
|
// amount is the tokens to delegate
|
|
cosmos.base.v1beta1.Coin amount = 3 [(gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgDelegateMintDepositResponse defines the Msg/MsgDelegateMintDeposit response type.
|
|
message MsgDelegateMintDepositResponse {}
|
|
|
|
// MsgWithdrawBurn removes staking derivatives from an earn vault and converts them back to a staking delegation.
|
|
message MsgWithdrawBurn {
|
|
// from is the owner of the earn vault to withdraw from
|
|
string from = 1 [(cosmos_proto.scalar) = "cosmos.AddressString"];
|
|
// validator is the address to select the derivative denom to withdraw
|
|
string validator = 2;
|
|
// amount is the staked token equivalent to withdraw
|
|
cosmos.base.v1beta1.Coin amount = 3 [(gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgWithdrawBurnResponse defines the Msg/MsgWithdrawBurn response type.
|
|
message MsgWithdrawBurnResponse {}
|
|
|
|
// MsgWithdrawBurnUndelegate removes staking derivatives from an earn vault, converts them to a staking delegation,
|
|
// then undelegates them from their validator.
|
|
message MsgWithdrawBurnUndelegate {
|
|
// from is the owner of the earn vault to withdraw from
|
|
string from = 1 [(cosmos_proto.scalar) = "cosmos.AddressString"];
|
|
// validator is the address to select the derivative denom to withdraw
|
|
string validator = 2;
|
|
// amount is the staked token equivalent to withdraw
|
|
cosmos.base.v1beta1.Coin amount = 3 [(gogoproto.nullable) = false];
|
|
}
|
|
|
|
// MsgWithdrawBurnUndelegateResponse defines the Msg/MsgWithdrawBurnUndelegate response type.
|
|
message MsgWithdrawBurnUndelegateResponse {}
|