mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 18:15:19 +00:00
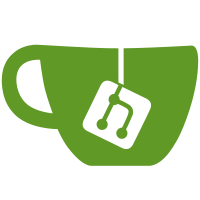
* Add 'InterestFactor' to CDP type (#734) * update cdp type to include interest factor * fix build * Add cdp accumulator methods (#735) * remame fees to interest * add accumulate interest method * add basic test * add note * address review comments * update tests * Add sync cdp interest method (#737) * remame fees to interest * add accumulate interest method * add basic test * add note * address review comments * update tests * remove old fee functions * add method to synchronize cdp interest * add multi-cdp tests * add test with many blocks * add test for interest getter * address review comments * calculate time difference then convert to seconds * fix: update collateral index when syncing interest * fix: differentiate between case when apy is zero and all fees are being rounded to zero * fix: round time difference properly * update cdp genesis state and migrations (#738) * remame fees to interest * add accumulate interest method * add basic test * add note * address review comments * update tests * remove old fee functions * add method to synchronize cdp interest * add multi-cdp tests * add test with many blocks * add test for interest getter * update cdp genesis state and migrations * address review comments * calculate time difference then convert to seconds * fix: update collateral index when syncing interest * fix: differentiate between case when apy is zero and all fees are being rounded to zero * fix: simplify add/remove/update collateral index * update genesis state to include total principal amounts * update migration * Delete kava-4-cdp-state-block-500000.json * Add cdp liquidations by external keeper (#750) * feat: split liquidations between external keepers and automated begin blocker * address review comments * USDX incentive accumulators (#752) * feat: split liquidations between external keepers and automated begin blocker * wip: refactor usdx minting incentives to use accumulators/hooks * wip: refactor usdx minting claim object * feat: use accumulators/hooks for usdx minting rewards * fix: get tests passing * fix: don't create claim objects unless that cdp type is eligable for rewards * add begin blocker * update client * cleanup comments/tests * update querier * address review comments * fix: check for division by zero * address review comments * run hook before interest is synced * Remove savings rate (#764) * remove savings rate * remove savings rate from debt param * update migrations * address review comments * Add usdx incentives calculation test (#765) * add usdx incentive calculation test * update reward calculation * add allowable error to test criteria * Update x/incentive/keeper/rewards_test.go Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> * fix: remove old fields from test genesis state Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com>
161 lines
4.2 KiB
Go
161 lines
4.2 KiB
Go
package cdp_test
|
|
|
|
import (
|
|
"strings"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/stretchr/testify/suite"
|
|
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
|
|
"github.com/kava-labs/kava/app"
|
|
"github.com/kava-labs/kava/x/cdp"
|
|
)
|
|
|
|
type GenesisTestSuite struct {
|
|
suite.Suite
|
|
|
|
ctx sdk.Context
|
|
keeper cdp.Keeper
|
|
}
|
|
|
|
func (suite *GenesisTestSuite) TestInvalidGenState() {
|
|
type args struct {
|
|
params cdp.Params
|
|
cdps cdp.CDPs
|
|
deposits cdp.Deposits
|
|
startingID uint64
|
|
debtDenom string
|
|
govDenom string
|
|
savingsRateDist sdk.Int
|
|
genAccumTimes cdp.GenesisAccumulationTimes
|
|
genTotalPrincipals cdp.GenesisTotalPrincipals
|
|
}
|
|
type errArgs struct {
|
|
expectPass bool
|
|
contains string
|
|
}
|
|
type genesisTest struct {
|
|
name string
|
|
args args
|
|
errArgs errArgs
|
|
}
|
|
testCases := []struct {
|
|
name string
|
|
args args
|
|
errArgs errArgs
|
|
}{
|
|
{
|
|
name: "empty debt denom",
|
|
args: args{
|
|
params: cdp.DefaultParams(),
|
|
cdps: cdp.CDPs{},
|
|
deposits: cdp.Deposits{},
|
|
debtDenom: "",
|
|
govDenom: cdp.DefaultGovDenom,
|
|
savingsRateDist: cdp.DefaultSavingsRateDistributed,
|
|
genAccumTimes: cdp.DefaultGenesisState().PreviousAccumulationTimes,
|
|
genTotalPrincipals: cdp.DefaultGenesisState().TotalPrincipals,
|
|
},
|
|
errArgs: errArgs{
|
|
expectPass: false,
|
|
contains: "debt denom invalid",
|
|
},
|
|
},
|
|
{
|
|
name: "empty gov denom",
|
|
args: args{
|
|
params: cdp.DefaultParams(),
|
|
cdps: cdp.CDPs{},
|
|
deposits: cdp.Deposits{},
|
|
debtDenom: cdp.DefaultDebtDenom,
|
|
govDenom: "",
|
|
savingsRateDist: cdp.DefaultSavingsRateDistributed,
|
|
genAccumTimes: cdp.DefaultGenesisState().PreviousAccumulationTimes,
|
|
genTotalPrincipals: cdp.DefaultGenesisState().TotalPrincipals,
|
|
},
|
|
errArgs: errArgs{
|
|
expectPass: false,
|
|
contains: "gov denom invalid",
|
|
},
|
|
},
|
|
{
|
|
name: "interest factor below one",
|
|
args: args{
|
|
params: cdp.DefaultParams(),
|
|
cdps: cdp.CDPs{},
|
|
deposits: cdp.Deposits{},
|
|
debtDenom: cdp.DefaultDebtDenom,
|
|
govDenom: cdp.DefaultGovDenom,
|
|
savingsRateDist: sdk.NewInt(100),
|
|
genAccumTimes: cdp.GenesisAccumulationTimes{cdp.NewGenesisAccumulationTime("bnb-a", time.Time{}, sdk.OneDec().Sub(sdk.SmallestDec()))},
|
|
genTotalPrincipals: cdp.DefaultGenesisState().TotalPrincipals,
|
|
},
|
|
errArgs: errArgs{
|
|
expectPass: false,
|
|
contains: "interest factor should be ≥ 1.0",
|
|
},
|
|
},
|
|
{
|
|
name: "negative total principal",
|
|
args: args{
|
|
params: cdp.DefaultParams(),
|
|
cdps: cdp.CDPs{},
|
|
deposits: cdp.Deposits{},
|
|
debtDenom: cdp.DefaultDebtDenom,
|
|
govDenom: cdp.DefaultGovDenom,
|
|
savingsRateDist: sdk.NewInt(100),
|
|
genAccumTimes: cdp.DefaultGenesisState().PreviousAccumulationTimes,
|
|
genTotalPrincipals: cdp.GenesisTotalPrincipals{cdp.NewGenesisTotalPrincipal("bnb-a", sdk.NewInt(-1))},
|
|
},
|
|
errArgs: errArgs{
|
|
expectPass: false,
|
|
contains: "total principal should be positive",
|
|
},
|
|
},
|
|
}
|
|
for _, tc := range testCases {
|
|
suite.Run(tc.name, func() {
|
|
gs := cdp.NewGenesisState(tc.args.params, tc.args.cdps, tc.args.deposits, tc.args.startingID,
|
|
tc.args.debtDenom, tc.args.govDenom, tc.args.genAccumTimes, tc.args.genTotalPrincipals)
|
|
err := gs.Validate()
|
|
if tc.errArgs.expectPass {
|
|
suite.Require().NoError(err)
|
|
} else {
|
|
suite.Require().Error(err)
|
|
suite.Require().True(strings.Contains(err.Error(), tc.errArgs.contains))
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func (suite *GenesisTestSuite) TestValidGenState() {
|
|
tApp := app.NewTestApp()
|
|
|
|
suite.NotPanics(func() {
|
|
tApp.InitializeFromGenesisStates(
|
|
NewPricefeedGenStateMulti(),
|
|
NewCDPGenStateMulti(),
|
|
)
|
|
})
|
|
|
|
cdpGS := NewCDPGenStateMulti()
|
|
gs := cdp.GenesisState{}
|
|
cdp.ModuleCdc.UnmarshalJSON(cdpGS["cdp"], &gs)
|
|
gs.CDPs = cdps()
|
|
gs.StartingCdpID = uint64(5)
|
|
appGS := app.GenesisState{"cdp": cdp.ModuleCdc.MustMarshalJSON(gs)}
|
|
suite.NotPanics(func() {
|
|
tApp.InitializeFromGenesisStates(
|
|
NewPricefeedGenStateMulti(),
|
|
appGS,
|
|
)
|
|
})
|
|
|
|
}
|
|
|
|
func TestGenesisTestSuite(t *testing.T) {
|
|
suite.Run(t, new(GenesisTestSuite))
|
|
}
|