mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
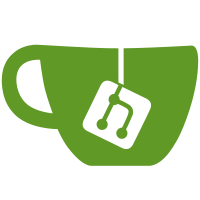
* ibc v3 upgrade * ibc no longer uses confio * add proofs proto for ibc/v3 * wip add ethermint module * update cosmos to 0.45.0 * add ethermint proto & bug fixes * remove todo * update docs * fix a number of bugs * minor comments update * fix breaking tests * Wrap bank keeper for EVM to convert decimals (#1154) * Add bankkeeper wrapper for evm * Remove agas from init-new-chain.sh, use ukava for evm_denom * Fix sdk.Coins conversion, require min 1 coin amount * Remove gas from init script idk how this happened lol * Remove debug logging stmt * Restore original init ukava amounts * Fix inplace coins conversion * Use evmtypes.BankKeeper interface insteadof banktypes * Add TestGetBalance * Add doc comments, remove temp actualAmt vars actualAmt vars replaced with inline calls to make it more clear that the converted value is being used, as opposed to accidentally reusing the raw EVM amt. * Add TestSetBalance * Add TestIdempotentConversion * Panic if converted coin from EVM is 0 This happens if a value is less than 1ukava * Deep copy coins instead of in place modification * Update test coins amount * Add panic tests for small EVM amounts * Use evmtypes.BankKeeper as NewEVMBankKeeper param * Tidy test setup * ensure sdk config is set when creating new apps * Respond EVM bank keeper GetBalance with SpendableCoins Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com> * further speed up docker builds * feat: restore previous keys add defaults, add eth flag (#1172) * feat: restore previous keys add defaults, add eth flag * remove outdated comment * fix: remove redundant flag default * evm bank keeper with akava handling * fix issues * add remaining tests * add emv module to app * add missing imports * clean up comments * wip akava keeper * evm keeper * fix genesis import * reduce module permissions * add bank keeper tests * cleanup tests * genesis tests * change defaults * add eth faucet key & fix issues * switch to kava ethermint * add a lot of tests * add balances invariant * add evm tests * Remove panic if Swagger disabled in config (#1155) (#1183) Co-authored-by: Derrick Lee <derrick@dlee.dev> * add invariant to catch any akava balance > 1 ukava * clarify name of balances invariant * connect invariants to app * fix evmbankkeeper akava issues * add spec for evmutil * remove zero balance accounts from state * minor adustments * update to ethermint 0.10.0 * fix eth ante * add missing godoc comment * Update x/evmutil/spec/01_concepts.md Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> * Update x/evmutil/spec/01_concepts.md Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> * Update ethermint to v0.12 (#1203) * update to ethermint v0.12.2 * use app.Options for new evm options * fix missed references to app.Options * use ethermint branch while waiting on upstream fix * evm migrations for tesnet alpha 2 (#1206) * update to ethermint v0.12.2 * use app.Options for new evm options * fix missed references to app.Options * use ethermint branch while waiting on upstream fix * add upgrade handler for evm-alpha testnet 2 * v17 migration setup + evm modules * refactor migrate states * x/feemarket migration * v17 migrations setup + evm modules migration (#1210) * v17 migration setup + evm modules * refactor migrate states * update gen time * fix: update genesis time in test output Co-authored-by: karzak <kjydavis3@gmail.com> * add savings module to app blockers Co-authored-by: Derrick Lee <derrick@dlee.dev> Co-authored-by: Nick DeLuca <nickdeluca08@gmail.com> Co-authored-by: rhuairahrighairigh <ruaridh.odonnell@gmail.com> Co-authored-by: Kevin Davis <karzak@users.noreply.github.com> Co-authored-by: Ruaridh <rhuairahrighairidh@users.noreply.github.com> Co-authored-by: karzak <kjydavis3@gmail.com>
273 lines
8.9 KiB
Protocol Buffer
273 lines
8.9 KiB
Protocol Buffer
syntax = "proto3";
|
|
package ethermint.evm.v1;
|
|
|
|
import "gogoproto/gogo.proto";
|
|
import "cosmos/base/query/v1beta1/pagination.proto";
|
|
import "google/api/annotations.proto";
|
|
import "ethermint/evm/v1/evm.proto";
|
|
import "ethermint/evm/v1/tx.proto";
|
|
import "google/protobuf/timestamp.proto";
|
|
|
|
option go_package = "github.com/tharsis/ethermint/x/evm/types";
|
|
|
|
// Query defines the gRPC querier service.
|
|
service Query {
|
|
// Account queries an Ethereum account.
|
|
rpc Account(QueryAccountRequest) returns (QueryAccountResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/account/{address}";
|
|
}
|
|
|
|
// CosmosAccount queries an Ethereum account's Cosmos Address.
|
|
rpc CosmosAccount(QueryCosmosAccountRequest)
|
|
returns (QueryCosmosAccountResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/cosmos_account/{address}";
|
|
}
|
|
|
|
// ValidatorAccount queries an Ethereum account's from a validator consensus
|
|
// Address.
|
|
rpc ValidatorAccount(QueryValidatorAccountRequest)
|
|
returns (QueryValidatorAccountResponse) {
|
|
option (google.api.http).get =
|
|
"/ethermint/evm/v1/validator_account/{cons_address}";
|
|
}
|
|
|
|
// Balance queries the balance of a the EVM denomination for a single
|
|
// EthAccount.
|
|
rpc Balance(QueryBalanceRequest) returns (QueryBalanceResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/balances/{address}";
|
|
}
|
|
|
|
// Storage queries the balance of all coins for a single account.
|
|
rpc Storage(QueryStorageRequest) returns (QueryStorageResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/storage/{address}/{key}";
|
|
}
|
|
|
|
// Code queries the balance of all coins for a single account.
|
|
rpc Code(QueryCodeRequest) returns (QueryCodeResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/codes/{address}";
|
|
}
|
|
|
|
// Params queries the parameters of x/evm module.
|
|
rpc Params(QueryParamsRequest) returns (QueryParamsResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/params";
|
|
}
|
|
|
|
// EthCall implements the `eth_call` rpc api
|
|
rpc EthCall(EthCallRequest) returns (MsgEthereumTxResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/eth_call";
|
|
}
|
|
|
|
// EstimateGas implements the `eth_estimateGas` rpc api
|
|
rpc EstimateGas(EthCallRequest) returns (EstimateGasResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/estimate_gas";
|
|
}
|
|
|
|
// TraceTx implements the `debug_traceTransaction` rpc api
|
|
rpc TraceTx(QueryTraceTxRequest) returns (QueryTraceTxResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/trace_tx";
|
|
}
|
|
|
|
// TraceBlock implements the `debug_traceBlockByNumber` and `debug_traceBlockByHash` rpc api
|
|
rpc TraceBlock(QueryTraceBlockRequest) returns (QueryTraceBlockResponse) {
|
|
option (google.api.http).get = "/ethermint/evm/v1/trace_block";
|
|
}
|
|
}
|
|
|
|
// QueryAccountRequest is the request type for the Query/Account RPC method.
|
|
message QueryAccountRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// address is the ethereum hex address to query the account for.
|
|
string address = 1;
|
|
}
|
|
|
|
// QueryAccountResponse is the response type for the Query/Account RPC method.
|
|
message QueryAccountResponse {
|
|
// balance is the balance of the EVM denomination.
|
|
string balance = 1;
|
|
// code hash is the hex-formatted code bytes from the EOA.
|
|
string code_hash = 2;
|
|
// nonce is the account's sequence number.
|
|
uint64 nonce = 3;
|
|
}
|
|
|
|
// QueryCosmosAccountRequest is the request type for the Query/CosmosAccount RPC
|
|
// method.
|
|
message QueryCosmosAccountRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// address is the ethereum hex address to query the account for.
|
|
string address = 1;
|
|
}
|
|
|
|
// QueryCosmosAccountResponse is the response type for the Query/CosmosAccount
|
|
// RPC method.
|
|
message QueryCosmosAccountResponse {
|
|
// cosmos_address is the cosmos address of the account.
|
|
string cosmos_address = 1;
|
|
// sequence is the account's sequence number.
|
|
uint64 sequence = 2;
|
|
// account_number is the account numbert
|
|
uint64 account_number = 3;
|
|
}
|
|
|
|
// QueryValidatorAccountRequest is the request type for the
|
|
// Query/ValidatorAccount RPC method.
|
|
message QueryValidatorAccountRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// cons_address is the validator cons address to query the account for.
|
|
string cons_address = 1;
|
|
}
|
|
|
|
// QueryValidatorAccountResponse is the response type for the
|
|
// Query/ValidatorAccount RPC method.
|
|
message QueryValidatorAccountResponse {
|
|
// account_address is the cosmos address of the account in bech32 format.
|
|
string account_address = 1;
|
|
// sequence is the account's sequence number.
|
|
uint64 sequence = 2;
|
|
// account_number is the account number
|
|
uint64 account_number = 3;
|
|
}
|
|
|
|
// QueryBalanceRequest is the request type for the Query/Balance RPC method.
|
|
message QueryBalanceRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// address is the ethereum hex address to query the balance for.
|
|
string address = 1;
|
|
}
|
|
|
|
// QueryBalanceResponse is the response type for the Query/Balance RPC method.
|
|
message QueryBalanceResponse {
|
|
// balance is the balance of the EVM denomination.
|
|
string balance = 1;
|
|
}
|
|
|
|
// QueryStorageRequest is the request type for the Query/Storage RPC method.
|
|
message QueryStorageRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
/// address is the ethereum hex address to query the storage state for.
|
|
string address = 1;
|
|
|
|
// key defines the key of the storage state
|
|
string key = 2;
|
|
}
|
|
|
|
// QueryStorageResponse is the response type for the Query/Storage RPC
|
|
// method.
|
|
message QueryStorageResponse {
|
|
// key defines the storage state value hash associated with the given key.
|
|
string value = 1;
|
|
}
|
|
|
|
// QueryCodeRequest is the request type for the Query/Code RPC method.
|
|
message QueryCodeRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// address is the ethereum hex address to query the code for.
|
|
string address = 1;
|
|
}
|
|
|
|
// QueryCodeResponse is the response type for the Query/Code RPC
|
|
// method.
|
|
message QueryCodeResponse {
|
|
// code represents the code bytes from an ethereum address.
|
|
bytes code = 1;
|
|
}
|
|
|
|
// QueryTxLogsRequest is the request type for the Query/TxLogs RPC method.
|
|
message QueryTxLogsRequest {
|
|
option (gogoproto.equal) = false;
|
|
option (gogoproto.goproto_getters) = false;
|
|
|
|
// hash is the ethereum transaction hex hash to query the logs for.
|
|
string hash = 1;
|
|
// pagination defines an optional pagination for the request.
|
|
cosmos.base.query.v1beta1.PageRequest pagination = 2;
|
|
}
|
|
|
|
// QueryTxLogs is the response type for the Query/TxLogs RPC method.
|
|
message QueryTxLogsResponse {
|
|
// logs represents the ethereum logs generated from the given transaction.
|
|
repeated Log logs = 1;
|
|
// pagination defines the pagination in the response.
|
|
cosmos.base.query.v1beta1.PageResponse pagination = 2;
|
|
}
|
|
|
|
// QueryParamsRequest defines the request type for querying x/evm parameters.
|
|
message QueryParamsRequest {}
|
|
|
|
// QueryParamsResponse defines the response type for querying x/evm parameters.
|
|
message QueryParamsResponse {
|
|
// params define the evm module parameters.
|
|
Params params = 1 [ (gogoproto.nullable) = false ];
|
|
}
|
|
|
|
// EthCallRequest defines EthCall request
|
|
message EthCallRequest {
|
|
// same json format as the json rpc api.
|
|
bytes args = 1;
|
|
// the default gas cap to be used
|
|
uint64 gas_cap = 2;
|
|
}
|
|
|
|
// EstimateGasResponse defines EstimateGas response
|
|
message EstimateGasResponse {
|
|
// the estimated gas
|
|
uint64 gas = 1;
|
|
}
|
|
|
|
// QueryTraceTxRequest defines TraceTx request
|
|
message QueryTraceTxRequest {
|
|
// msgEthereumTx for the requested transaction
|
|
MsgEthereumTx msg = 1;
|
|
// transaction index
|
|
uint64 tx_index = 2;
|
|
// TraceConfig holds extra parameters to trace functions.
|
|
TraceConfig trace_config = 3;
|
|
// the predecessor transactions included in the same block
|
|
// need to be replayed first to get correct context for tracing.
|
|
repeated MsgEthereumTx predecessors = 4;
|
|
// block number of requested transaction
|
|
int64 block_number = 5;
|
|
// block hex hash of requested transaction
|
|
string block_hash = 6;
|
|
// block time of requested transaction
|
|
google.protobuf.Timestamp block_time = 7 [(gogoproto.nullable) = false, (gogoproto.stdtime) = true];
|
|
}
|
|
|
|
// QueryTraceTxResponse defines TraceTx response
|
|
message QueryTraceTxResponse {
|
|
// response serialized in bytes
|
|
bytes data = 1;
|
|
}
|
|
|
|
// QueryTraceBlockRequest defines TraceTx request
|
|
message QueryTraceBlockRequest {
|
|
// txs messages in the block
|
|
repeated MsgEthereumTx txs = 1;
|
|
// TraceConfig holds extra parameters to trace functions.
|
|
TraceConfig trace_config = 3;
|
|
// block number
|
|
int64 block_number = 5;
|
|
// block hex hash
|
|
string block_hash = 6;
|
|
// block time
|
|
google.protobuf.Timestamp block_time = 7 [(gogoproto.nullable) = false, (gogoproto.stdtime) = true];
|
|
}
|
|
|
|
// QueryTraceBlockResponse defines TraceBlock response
|
|
message QueryTraceBlockResponse {
|
|
bytes data = 1;
|
|
}
|
|
|