mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
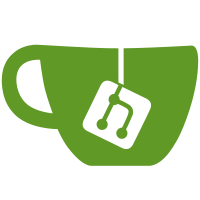
* add minAmount, maxAmount * update kava-3 params for compile * fix migration script * update to mainnet params * remove height span validation for incoming swaps * update to sdk.Int, set lock to 220 * update lock range to [220, 270] * update bep3 module docs * update MsgClaim's ValidateBasic * update test comments
96 lines
2.7 KiB
Go
96 lines
2.7 KiB
Go
package keeper
|
|
|
|
import (
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
sdkerrors "github.com/cosmos/cosmos-sdk/types/errors"
|
|
|
|
"github.com/kava-labs/kava/x/bep3/types"
|
|
)
|
|
|
|
// GetParams returns the total set of bep3 parameters.
|
|
func (k Keeper) GetParams(ctx sdk.Context) (params types.Params) {
|
|
k.paramSubspace.GetParamSet(ctx, ¶ms)
|
|
return params
|
|
}
|
|
|
|
// SetParams sets the bep3 parameters to the param space.
|
|
func (k Keeper) SetParams(ctx sdk.Context, params types.Params) {
|
|
k.paramSubspace.SetParamSet(ctx, ¶ms)
|
|
}
|
|
|
|
// GetBnbDeputyAddress returns the Bnbchain's deputy address
|
|
func (k Keeper) GetBnbDeputyAddress(ctx sdk.Context) sdk.AccAddress {
|
|
params := k.GetParams(ctx)
|
|
return params.BnbDeputyAddress
|
|
}
|
|
|
|
// GetBnbDeputyFixedFee returns the deputy's fixed fee
|
|
func (k Keeper) GetBnbDeputyFixedFee(ctx sdk.Context) sdk.Int {
|
|
params := k.GetParams(ctx)
|
|
return params.BnbDeputyFixedFee
|
|
}
|
|
|
|
// GetMinAmount returns the minimum amount
|
|
func (k Keeper) GetMinAmount(ctx sdk.Context) sdk.Int {
|
|
params := k.GetParams(ctx)
|
|
return params.MinAmount
|
|
}
|
|
|
|
// GetMaxAmount returns the maximum amount
|
|
func (k Keeper) GetMaxAmount(ctx sdk.Context) sdk.Int {
|
|
params := k.GetParams(ctx)
|
|
return params.MaxAmount
|
|
}
|
|
|
|
// GetMinBlockLock returns the minimum block lock
|
|
func (k Keeper) GetMinBlockLock(ctx sdk.Context) uint64 {
|
|
params := k.GetParams(ctx)
|
|
return params.MinBlockLock
|
|
}
|
|
|
|
// GetMaxBlockLock returns the maximum block lock
|
|
func (k Keeper) GetMaxBlockLock(ctx sdk.Context) uint64 {
|
|
params := k.GetParams(ctx)
|
|
return params.MaxBlockLock
|
|
}
|
|
|
|
// GetAssets returns a list containing all supported assets
|
|
func (k Keeper) GetAssets(ctx sdk.Context) (types.AssetParams, bool) {
|
|
params := k.GetParams(ctx)
|
|
return params.SupportedAssets, len(params.SupportedAssets) > 0
|
|
}
|
|
|
|
// GetAssetByDenom returns an asset by its denom
|
|
func (k Keeper) GetAssetByDenom(ctx sdk.Context, denom string) (types.AssetParam, bool) {
|
|
params := k.GetParams(ctx)
|
|
for _, asset := range params.SupportedAssets {
|
|
if asset.Denom == denom {
|
|
return asset, true
|
|
}
|
|
}
|
|
return types.AssetParam{}, false
|
|
}
|
|
|
|
// GetAssetByCoinID returns an asset by its denom
|
|
func (k Keeper) GetAssetByCoinID(ctx sdk.Context, coinID int) (types.AssetParam, bool) {
|
|
params := k.GetParams(ctx)
|
|
for _, asset := range params.SupportedAssets {
|
|
if asset.CoinID == coinID {
|
|
return asset, true
|
|
}
|
|
}
|
|
return types.AssetParam{}, false
|
|
}
|
|
|
|
// ValidateLiveAsset checks if an asset is both supported and active
|
|
func (k Keeper) ValidateLiveAsset(ctx sdk.Context, coin sdk.Coin) error {
|
|
asset, found := k.GetAssetByDenom(ctx, coin.Denom)
|
|
if !found {
|
|
return sdkerrors.Wrap(types.ErrAssetNotSupported, coin.Denom)
|
|
}
|
|
if !asset.Active {
|
|
return sdkerrors.Wrap(types.ErrAssetNotActive, asset.Denom)
|
|
}
|
|
return nil
|
|
}
|