mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 22:57:32 +00:00
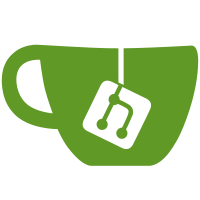
* first pass * fix bid amount calculation * untested refactor of sim ops and genesis * refactor operations and fix auction bug * add param changes and genesis * address minor TODO * add first draft of invariants * improve param generation * complete invariants * fix genesis tests * log no-op better * small fixes * add missed comma Co-authored-by: John Maheswaran <john@kava.io>
47 lines
1.6 KiB
Go
47 lines
1.6 KiB
Go
package simulation
|
|
|
|
import (
|
|
"fmt"
|
|
"math/rand"
|
|
|
|
"github.com/cosmos/cosmos-sdk/x/simulation"
|
|
|
|
"github.com/kava-labs/kava/x/auction/types"
|
|
)
|
|
|
|
// ParamChanges defines the parameters that can be modified by param change proposals
|
|
// on the simulation
|
|
func ParamChanges(r *rand.Rand) []simulation.ParamChange {
|
|
// Note: params are encoded to JSON before being stored in the param store. These param changes
|
|
// update the raw values in the store so values need to be JSON. This is why values that are represented
|
|
// as strings in JSON (such as time.Duration) have the escaped quotes.
|
|
// TODO should we encode the values properly with ModuleCdc.MustMarshalJSON()?
|
|
return []simulation.ParamChange{
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyBidDuration), "",
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("\"%d\"", GenBidDuration(r))
|
|
},
|
|
),
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyMaxAuctionDuration), "",
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("\"%d\"", GenMaxAuctionDuration(r))
|
|
},
|
|
),
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyIncrementCollateral), "",
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("\"%d\"", GenIncrementCollateral(r))
|
|
},
|
|
),
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyIncrementDebt), "",
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("\"%d\"", GenIncrementDebt(r))
|
|
},
|
|
),
|
|
simulation.NewSimParamChange(types.ModuleName, string(types.KeyIncrementSurplus), "",
|
|
func(r *rand.Rand) string {
|
|
return fmt.Sprintf("\"%d\"", GenIncrementSurplus(r))
|
|
},
|
|
),
|
|
}
|
|
}
|