mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-11-10 10:05:18 +00:00
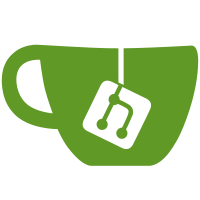
* comments from review Co-authored-by: Sunny Aggarwal <sunnya97@protonmail.ch> Co-authored-by: jmahess <maheswaran@google.com> Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> * add vote methods * add draft new param change permission * add and update tests * rename ParamChangePermission * account for perms becoming invalid at a later time * add debtParam to permission * add bep3 AssetParam to permissions * add pricefeed Markets to permission * add upgrade permission * move proposal passing to the begin blocker * fix iteration bug Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com> * address todos and audit comments * add proposal examples * refactor handler to be easier to read * address review comments * update comments Co-authored-by: Kevin Davis <kjydavis3@gmail.com> Co-authored-by: Sunny Aggarwal <sunnya97@protonmail.ch> Co-authored-by: jmahess <maheswaran@google.com> Co-authored-by: Alexander Bezobchuk <alexanderbez@users.noreply.github.com> Co-authored-by: Federico Kunze <31522760+fedekunze@users.noreply.github.com>
53 lines
1.6 KiB
Go
53 lines
1.6 KiB
Go
package committee
|
|
|
|
import (
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
sdkerrors "github.com/cosmos/cosmos-sdk/types/errors"
|
|
govtypes "github.com/cosmos/cosmos-sdk/x/gov/types"
|
|
)
|
|
|
|
func NewProposalHandler(k Keeper) govtypes.Handler {
|
|
return func(ctx sdk.Context, content govtypes.Content) error {
|
|
switch c := content.(type) {
|
|
case CommitteeChangeProposal:
|
|
return handleCommitteeChangeProposal(ctx, k, c)
|
|
case CommitteeDeleteProposal:
|
|
return handleCommitteeDeleteProposal(ctx, k, c)
|
|
|
|
default:
|
|
return sdkerrors.Wrapf(sdkerrors.ErrUnknownRequest, "unrecognized %s proposal content type: %T", ModuleName, c)
|
|
}
|
|
}
|
|
}
|
|
|
|
func handleCommitteeChangeProposal(ctx sdk.Context, k Keeper, committeeProposal CommitteeChangeProposal) error {
|
|
if err := committeeProposal.ValidateBasic(); err != nil {
|
|
return sdkerrors.Wrap(ErrInvalidPubProposal, err.Error())
|
|
}
|
|
|
|
// Remove all committee's ongoing proposals
|
|
proposals := k.GetProposalsByCommittee(ctx, committeeProposal.NewCommittee.ID)
|
|
for _, p := range proposals {
|
|
k.DeleteProposalAndVotes(ctx, p.ID)
|
|
}
|
|
|
|
// update/create the committee
|
|
k.SetCommittee(ctx, committeeProposal.NewCommittee)
|
|
return nil
|
|
}
|
|
|
|
func handleCommitteeDeleteProposal(ctx sdk.Context, k Keeper, committeeProposal CommitteeDeleteProposal) error {
|
|
if err := committeeProposal.ValidateBasic(); err != nil {
|
|
return sdkerrors.Wrap(ErrInvalidPubProposal, err.Error())
|
|
}
|
|
|
|
// Remove all committee's ongoing proposals
|
|
proposals := k.GetProposalsByCommittee(ctx, committeeProposal.CommitteeID)
|
|
for _, p := range proposals {
|
|
k.DeleteProposalAndVotes(ctx, p.ID)
|
|
}
|
|
|
|
k.DeleteCommittee(ctx, committeeProposal.CommitteeID)
|
|
return nil
|
|
}
|