mirror of
https://github.com/0glabs/0g-chain.git
synced 2024-09-20 14:47:31 +00:00
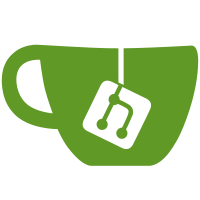
* add get set methods for swap reward indexes * add get set methods for swap accrual time * tidy up location of multi periods * add swap reward periods to params * add initial legacy types for incentive * minor refactor of migration code * add incentive migration for swap params * minor incentive test refactors * add math methods to RewardIndexes * add keeper method to increment global indexes * add swap keeper to incentive keeper * indicate if pool shares were found or not * add accumulator to compute new rewards each block * accumulate swap rewards globally * remove unecessary keeper method * expand doc comments on accumulator methods * test precision not lost in accumulation * minor fixes from merge * rename storeGlobalDelegatorFactor to match others * fix migration from merge * fix bug in app setup * fix accumulation bug when starting with no state * rename swap files to match others * add swap accumulation times to genesis * remove old migration refactor * minor updates to spec * add high level description of how rewards work
51 lines
1.4 KiB
Go
51 lines
1.4 KiB
Go
package migrate
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/cosmos/cosmos-sdk/codec"
|
|
"github.com/cosmos/cosmos-sdk/server"
|
|
sdk "github.com/cosmos/cosmos-sdk/types"
|
|
"github.com/cosmos/cosmos-sdk/version"
|
|
"github.com/spf13/cobra"
|
|
tmtypes "github.com/tendermint/tendermint/types"
|
|
|
|
"github.com/kava-labs/kava/migrate/v0_15"
|
|
)
|
|
|
|
// MigrateGenesisCmd returns a command to execute genesis state migration.
|
|
func MigrateGenesisCmd(_ *server.Context, cdc *codec.Codec) *cobra.Command {
|
|
cmd := &cobra.Command{
|
|
Use: "migrate [genesis-file]",
|
|
Short: "Migrate genesis file from kava v0.14 to v0.15",
|
|
Long: "Migrate the source genesis into the current version, sorts it, and print to STDOUT.",
|
|
Example: fmt.Sprintf(`%s migrate /path/to/genesis.json`, version.ServerName),
|
|
Args: cobra.ExactArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
|
|
importGenesis := args[0]
|
|
genDoc, err := tmtypes.GenesisDocFromFile(importGenesis)
|
|
if err != nil {
|
|
return fmt.Errorf("failed to read genesis document from file %s: %w", importGenesis, err)
|
|
}
|
|
|
|
newGenDoc := v0_15.Migrate(*genDoc)
|
|
|
|
bz, err := cdc.MarshalJSONIndent(newGenDoc, "", " ")
|
|
if err != nil {
|
|
return fmt.Errorf("failed to marshal genesis doc: %w", err)
|
|
}
|
|
|
|
sortedBz, err := sdk.SortJSON(bz)
|
|
if err != nil {
|
|
return fmt.Errorf("failed to sort JSON genesis doc: %w", err)
|
|
}
|
|
|
|
fmt.Println(string(sortedBz))
|
|
return nil
|
|
},
|
|
}
|
|
|
|
return cmd
|
|
}
|